auto-shorts
The AI-powered short video creator
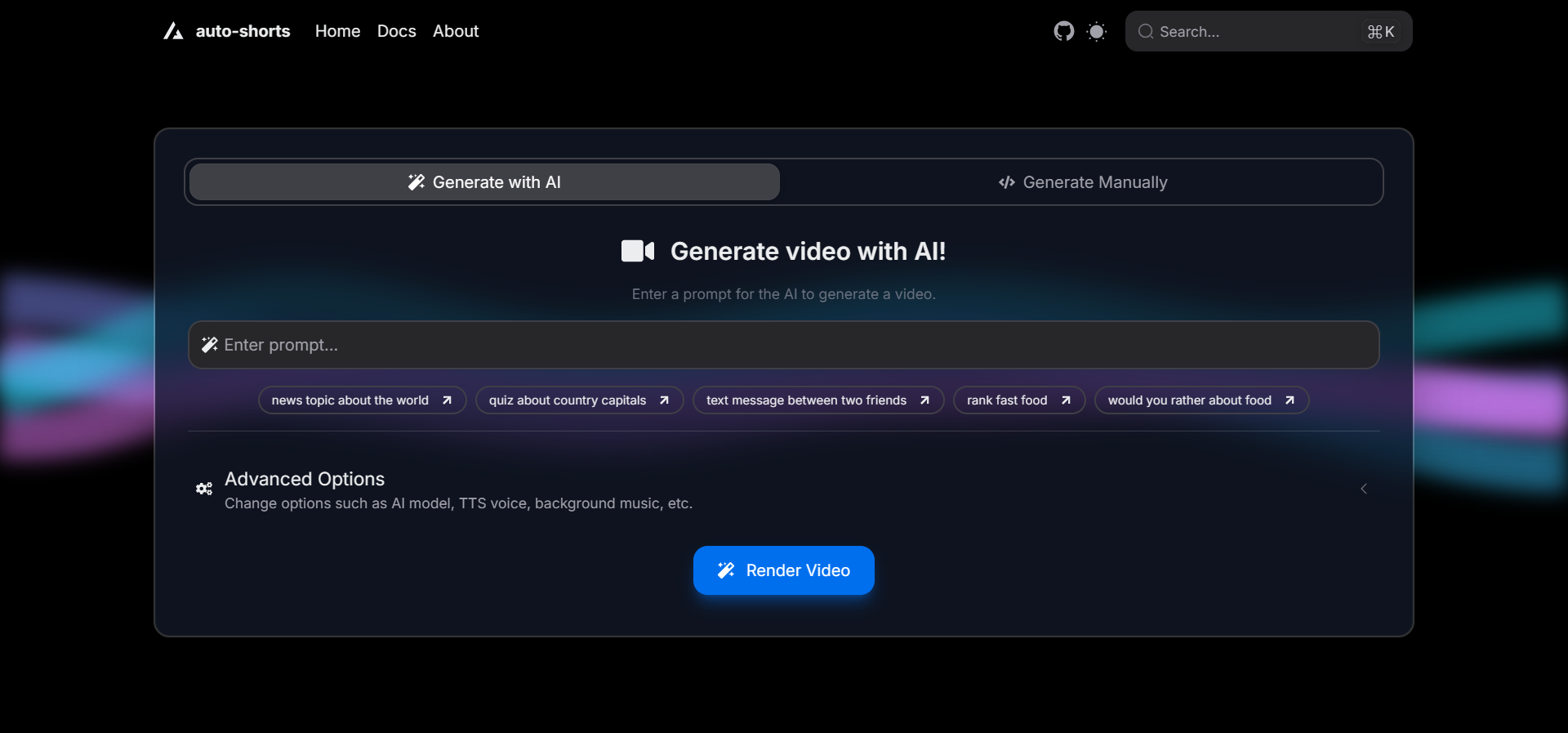
Easy to Use
auto-shorts is designed to be easy to use, with a simple and intuitive UI.
Generate any type of short
auto-shorts can generate any type of short, from simple to complex. Read the docs to learn more.
Use CLI, API, or Web Interface
auto-shorts can be used in multiple ways, including CLI, API (JS/TS), or Web Interface.